You can use either OutputStream or Writer class in Java to write data to a file in Java. For example, you can use a combination of FileWriter and BufferedWriter to write text content into a text file in Java. If you want to write raw bytes consider using FileOutputStream class. Just remember that InputStream is used to read data and OutputStream is used to write data to file or socket. You can write anything to file e.g. String, integer, float values etc. Java provides DataOutputStream to write different data type directly into file e.g. writeInt() to write integer values, writeFloat() to write floating point values into file and writeUTF() to write String into File. BufferedWriter, like its counterpart BufferedReader, allows you to perform buffered IO, which can drastically improve performance while reading large files.
Read more »mardi 30 juin 2015
Difference between Dependency Injection and Factory Pattern in Java
Posted on 05:14
by Malik korrich
TL;DR Main difference between dependency injection and factory pattern is that in the case of former dependency is provided by the third party (framework or container) while in the case of later dependency is acquired by client class itself. Another key difference between them is that use of dependency injection result in loosely coupled design but the use of factory pattern create a tight coupling between factory and classes which are dependent on the product created by the factory. Though both Dependency Injection and Factory pattern look similar in a sense that both creates an instance of a class, and also promotes interface driven programming rather than hard coding implementation class; But, there are some subtle differences between Factory pattern and dependency injection pattern.
Read more »samedi 27 juin 2015
2 Ways to check If String is Palindrome in Java? Recursion and Loop
Posted on 20:53
by Malik korrich
A String is said to be Palindrome if it is equal to itself in reverse order. You can use this logic to check if String is Palindrome or not. There are two common ways to find if a given String is Palindrome or not in Java, first by using for loop, also known as iterative algorithm and second by using recursion, also known as recursive algorithm. The crux of this problem lies in how do you reverse String in Java? because once you have the String in reverse order, problem reduced to just comparing itself with the reversed String. If both are equal then given String is Palindrome otherwise it's not. Also whether your solution is iterative or recursive will also determine by implementing this logic. If you reverse String using for loop then it become an iterative solution and if you reverse String using recursion then it become a recursive solution. In general, recursive solution are short, readable and more intuitive but subject to StackOverFlowError and that's why not advised to be used in production system. You should always be using iterative solution in production, unless your programming language supports tail recursion optimization e.g. Scala which eliminates risk of StackOverFlowError by internally converting a recursive solution to an iterative one. If you are doing this exercise as part of your Interview preparation then I suggest you to take a look at Cracking the Coding Interview: 150 Programming Questions and Solutions, as title says it contains 150 good questions based upon different topics e.g. String, array, linked list, binary tree, networking etc. A good book for preparing both Java and C++ interview.
Read more »java.lang.NoClassDefFoundError: org/dom4j/DocumentException [Solution]
Posted on 07:29
by Malik korrich
Exception in thread "main" java.lang.NoClassDefFoundError: org/dom4j/DocumentException comes when your program is using DOM4j library but necessary JAR is not present. This error can also come when you are indirectly using DOM4j library e.g. when you use Apache POI library to read XLSX file in Java, this library needs dom4j.jar in your classpath. Not just this one but there are several other libraries which use this JAR internally, if you are using any of them but don't have this JAR then your program will compile fine but fail at runtime because JVM will try to load this class but will not be able to find it on the classpath. Some curious developers might ask, why it didn't fail during compile time if JAR was not present there? Well, the reason for that is that your code might not be using any class file directly from the dom4j.jar file.
Read more »vendredi 26 juin 2015
java.lang.ClassNotFoundException: org.postgresql.Driver - Cause and Solution
Posted on 08:01
by Malik korrich
java.lang.ClassNotFoundException: org.postgresql.Driver error comes when you are trying to connect to a PostgreSQL database from Java program but Java ClassLoader is not able to find the Driver class "org.postgresql.Driver" required to make the connection. Usually this class is find in PostgreSQL JDBC driver JAR e.g. postgresql-9.4-1201.jdbc41.jar, which is required to connect PostgreSQL server version greater than 9.3 from JDK 1.7 or JDK 1.8, the exact JAR depends upon your PostgreSQL server version, the Java version you are running and JDBC version your are using. Now your problem could be either you don't have that PostgreSQL JDBC driver JAR in your machine or the JAR is not in your classpath, or you might be battling with some classpath intricacies. If you don't have this JAR, then solution of "java.lang.ClassNotFoundException: org.postgresql.Driver" is simple, just download it from PostgreSQL site. Make sure you download the correct version of JDBC driver based upon the PostgreSQL server you are connecting and JVM version of your machine. Once you download that, just put in the lib directory of your Java application e.g. WEB-INF/lib if you are connecting to PostgreSQL from Java Web Application. If you are running in Tomcat, then you can alternatively also put this in tomcat/lib directory, but beware of the difference in the application dependency in WEB-INF/lib or in tomcat/lib. You can also take a look at Practical database programming with Java book by Ying Bai. This books is a comprehensive guide of how to use JDBC in Java to connect to different databases. You will learn right ways of doing things with respect to Java and database.
Read more »2 Ways to Read a Text File in Java 6
Posted on 04:47
by Malik korrich
You can read a text file in Java 6 by using BufferedReader or Scanner class. Both classes provide convenient methods to read a text file line by line e.g. Scanner provides nextLine() method and BufferedReader provides readLine() method. If you are reading a binary file, you can use use FileInputStream. By the way, when you are reading text data, you also need to provide character encoding, if you don't then platform's default character encoding is used. In Java IO, streams like InputStream are used to read bytes and Readers like FileReader are used to read character data. BufferedReader is the traditional way to read data because it reads file buffer by buffer instead of character by character, so it's more efficient if you are reading large files. BufferedReader is also there from JDK 1 itself while Scanner was added to Java 5.
Read more »jeudi 25 juin 2015
Remove "Ads by PopShopCoupon" Adware (Uninstall Guide)
Posted on 10:34
by Malik korrich
Okay, so by now, you are probably well aware of "Ads by PopShopCoupon" advertisements and just what PopShopCoupon adware is. But do you know exactly what damage it can do to your computer and your user experience? We are sure that if you have been infected by a nastier strain of this adware then you are in complete agreement with me as to just what a nightmare it can be to deal with.
This adware might have a reputation as a bit of a softy in the world of malicious software because it 'only' displays ads by PopShopCoupon on your computer screen but that doesn't mean you should look the other way if you have been infected by it – or ignore its existence altogether. At the very least, it can be insanely irritating!
And although it is certainly possible to turn a blind eye to some types of adware, there is another variety which is extremely intrusive, both in front of, and behind the scenes.
What does adware look like?
The types of adverts that are easier to ignore are simply just ads by PopShopCoupon displayed on your screen, usually at the edges, when you are online. It is fairly easy to ignore advert links such as the ones that Google use, or banner adverts, but less forgivable are the pop-up or pop-under windows which will have you gritting your teeth in frustration as they constantly disrupt you while you are online.
If you've ever had pop-up adware on your computer you will know what a waste of time and energy it is as you battle to close the ads; clicking on the little red 'x' in the corner of the box to make them go away - only to have them pop back up again instantly.
How did PopShopCoupon adware get on to your computer the first place?
It is highly likely that just before you noticed the adware that you downloaded something: a TV show, an app or an upgrade of an existing program you have installed. Because PopShopCoupon adware is usually bundled with free programs and downloads it is highly likely that if you've executed a file or downloaded something else, adware will have snuck in through the backdoor that way.
What else can PopShopCoupon adware do?
This adware is an extreme form of advertising and is far better placed to manipulate us than print or traditional adverts ever could. And this manifests itself in a number of ways. It doesn't just show you adverts; it also spies on you so that the program's developer can tailor the adverts that you see towards your interests. And it does this by monitoring which websites you visit and then looking at the goods or services that you look at on those websites. A component installed by the adware collects this information and sends it back to the programmer. They can then increase your chances of clicking on the ad (and increase their chances of making a quick buck) by making sure that the adverts you see are more akin to your internet searches.
Adware is a real pain, so protect yourself by installing a decent anti-malware program today. If it's already too late and your computer is infected with this adware and its relentless "Ads by PopShopCoupon" advertisements, please follow the steps on the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove PopShopCoupon related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
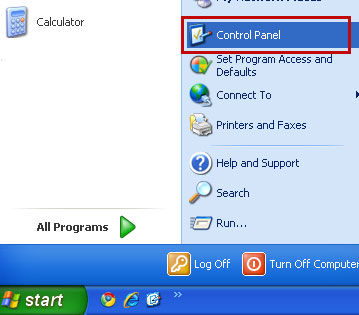
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
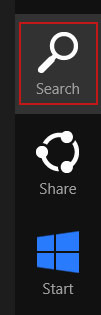
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
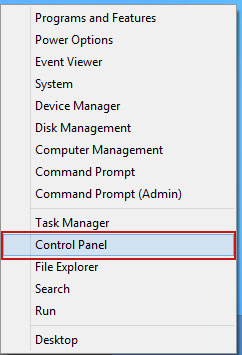
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
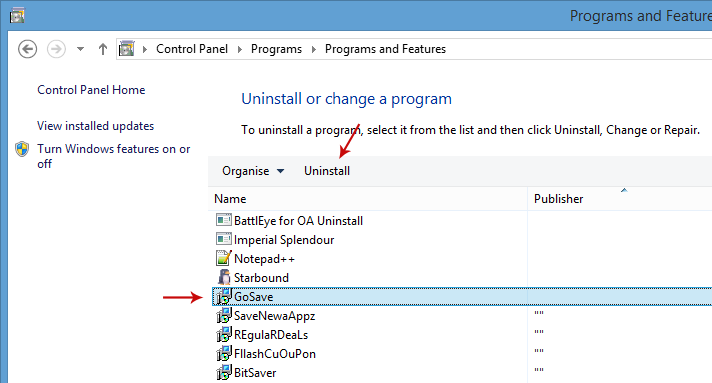
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove PopShopCoupon related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
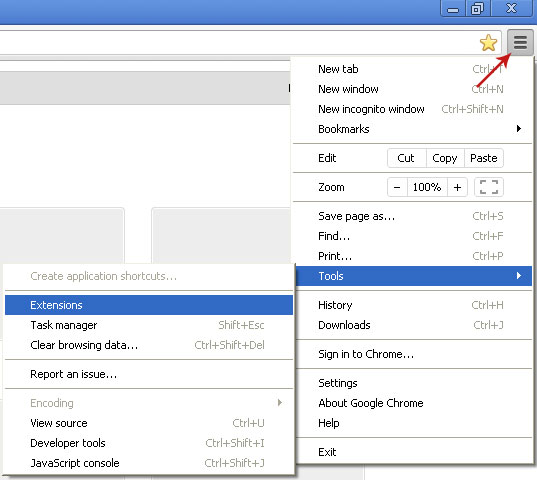
2. Click on the trashcan icon to remove PopShopCoupon, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove PopShopCoupon related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
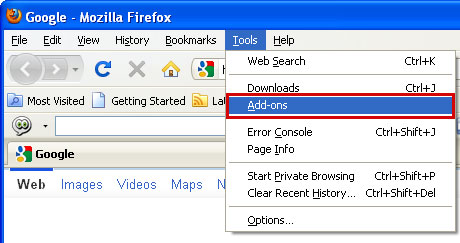
2. Select Extensions. Click Remove button to remove PopShopCoupon, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove PopShopCoupon related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
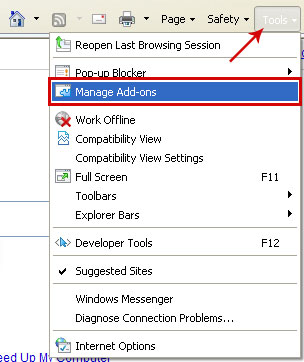
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
This adware might have a reputation as a bit of a softy in the world of malicious software because it 'only' displays ads by PopShopCoupon on your computer screen but that doesn't mean you should look the other way if you have been infected by it – or ignore its existence altogether. At the very least, it can be insanely irritating!
And although it is certainly possible to turn a blind eye to some types of adware, there is another variety which is extremely intrusive, both in front of, and behind the scenes.
What does adware look like?
The types of adverts that are easier to ignore are simply just ads by PopShopCoupon displayed on your screen, usually at the edges, when you are online. It is fairly easy to ignore advert links such as the ones that Google use, or banner adverts, but less forgivable are the pop-up or pop-under windows which will have you gritting your teeth in frustration as they constantly disrupt you while you are online.
If you've ever had pop-up adware on your computer you will know what a waste of time and energy it is as you battle to close the ads; clicking on the little red 'x' in the corner of the box to make them go away - only to have them pop back up again instantly.
How did PopShopCoupon adware get on to your computer the first place?
It is highly likely that just before you noticed the adware that you downloaded something: a TV show, an app or an upgrade of an existing program you have installed. Because PopShopCoupon adware is usually bundled with free programs and downloads it is highly likely that if you've executed a file or downloaded something else, adware will have snuck in through the backdoor that way.
What else can PopShopCoupon adware do?
This adware is an extreme form of advertising and is far better placed to manipulate us than print or traditional adverts ever could. And this manifests itself in a number of ways. It doesn't just show you adverts; it also spies on you so that the program's developer can tailor the adverts that you see towards your interests. And it does this by monitoring which websites you visit and then looking at the goods or services that you look at on those websites. A component installed by the adware collects this information and sends it back to the programmer. They can then increase your chances of clicking on the ad (and increase their chances of making a quick buck) by making sure that the adverts you see are more akin to your internet searches.
Adware is a real pain, so protect yourself by installing a decent anti-malware program today. If it's already too late and your computer is infected with this adware and its relentless "Ads by PopShopCoupon" advertisements, please follow the steps on the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
"Ads by PopShopCoupon" Removal Guide:
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove PopShopCoupon related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
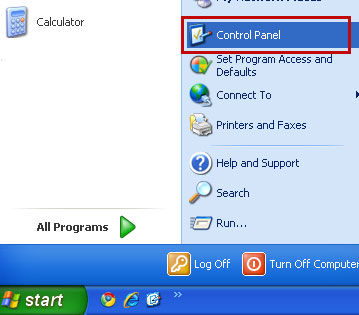
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
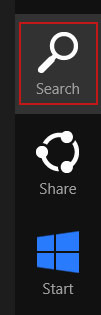
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
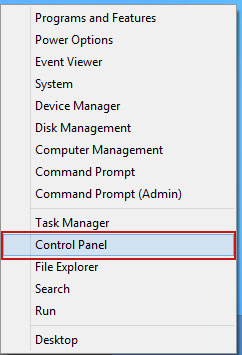
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
- PopShopCoupon
- GoSave
- Active Discount
- AdCoupon
- and any other recently installed application
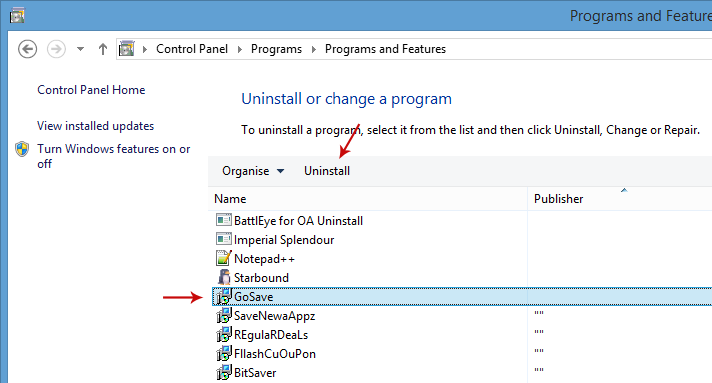
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove PopShopCoupon related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
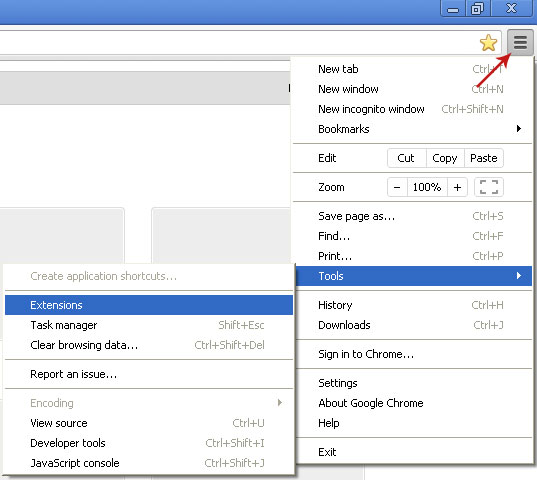
2. Click on the trashcan icon to remove PopShopCoupon, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove PopShopCoupon related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
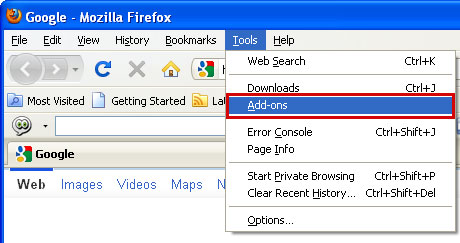
2. Select Extensions. Click Remove button to remove PopShopCoupon, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove PopShopCoupon related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
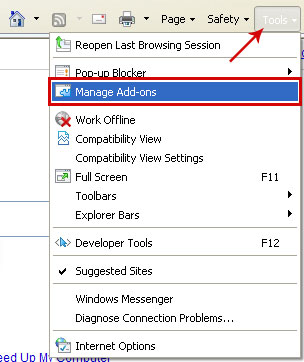
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
5 Websites to Learn SQL Online for FREE
Posted on 07:26
by Malik korrich
SQL is one of the most important skill for any programmer be it a Java, C++, PHP or Ruby developer. Almost 95% of the Java applications uses relational database in their back-end and almost all web applications uses database. In recent years, one of the most common way to learn any programming skill is online, at your comfort of office or home and SQL is no different. Learning SQL online has another advantage of quick head start because you don't need to install database and create tables to write some SELECT queries. Once you start writing queries and seeing result, you feel that confidence needed to go to next level, which involves downloading and installing free copy of popular databases e.g. MySQL, SQL Server or Oracle in your machine. From my experience I can say that SQL is easy to learn but difficult to master. You can start writing SQL queries in about an hour or so, but when it comes to write queries to solve real time requirements or for reporting purpose, its not that easy. Practicing SQL online on sites like SQLZoo or SQLFiddle will help you to keep yourself up-to-date. You can also take help from some good SQL books like Head First SQL if you are beginner, head first way is one of the best way to learn SQL.
Read more »mardi 23 juin 2015
Remove "Ads by Coupon Titan" Adware (Uninstall Guide)
Posted on 12:06
by Malik korrich
Coupon Titan adware, or advertising supported software, as it is otherwise known is a type of malware (or malicious software) that displays "Ads by Coupon Titan" advertisements on your web browser. But what is adware, is it really worth getting worried about, and how do you know if you've been infected by it?
Coupon Titan IS worth being concerned about because it can have a more serious knock on effect than many people give it credit for but the one slight thing it does have in its favour is that it is pretty easy to tell if you have been infected by it. Adware's strongest point, luckily for us, is not subtlety, which means that it is quick and easy to identify ads by Coupon Titan – giving you a good heads up that you should take steps to remove it, and then protect your computer from being compromised again in the future.
How can I tell if I have this adware on my computer or other device?
Whether you are using a PC or laptop, if you have been infected by this adware then you will see numerous by Coupon Titan pop-up windows, banner adverts, and even pop-under windows (ones that hide behind the screen you currently have open). This in itself pretty much gives the game away, but there are a few other indicators that you are currently undergoing an infestation of adware.
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove Coupon Titan related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
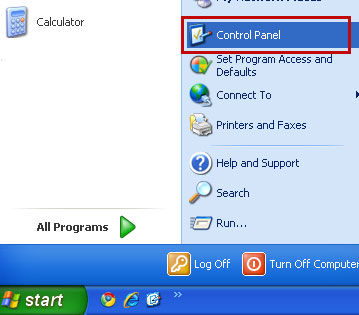
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
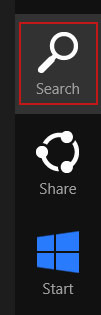
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
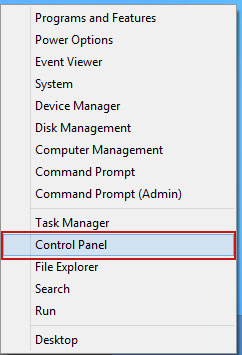
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
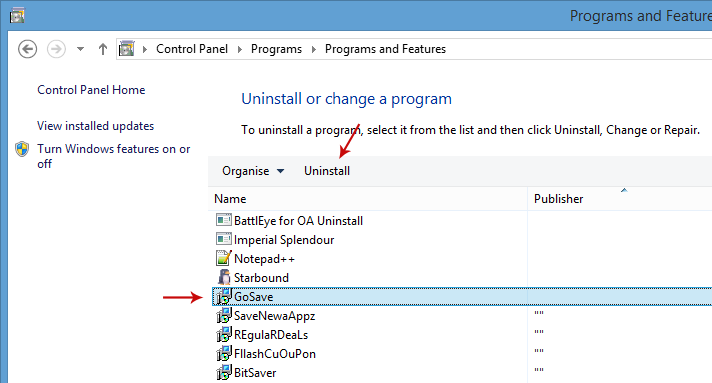
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove Coupon Titan related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
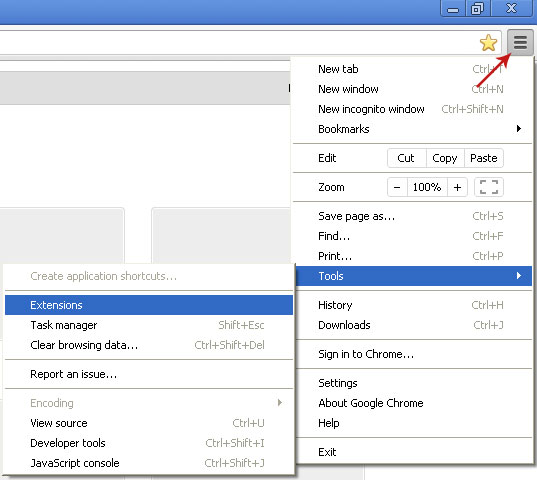
2. Click on the trashcan icon to remove Coupon Titan, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove Coupon Titan related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
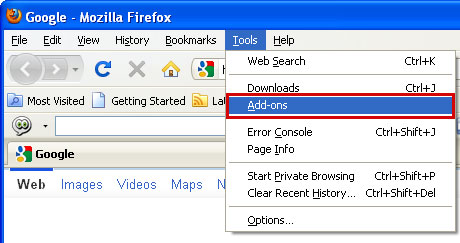
2. Select Extensions. Click Remove button to remove Coupon Titan, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove Coupon Titan related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
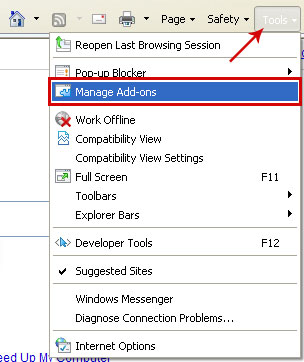
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
Coupon Titan IS worth being concerned about because it can have a more serious knock on effect than many people give it credit for but the one slight thing it does have in its favour is that it is pretty easy to tell if you have been infected by it. Adware's strongest point, luckily for us, is not subtlety, which means that it is quick and easy to identify ads by Coupon Titan – giving you a good heads up that you should take steps to remove it, and then protect your computer from being compromised again in the future.
How can I tell if I have this adware on my computer or other device?
Whether you are using a PC or laptop, if you have been infected by this adware then you will see numerous by Coupon Titan pop-up windows, banner adverts, and even pop-under windows (ones that hide behind the screen you currently have open). This in itself pretty much gives the game away, but there are a few other indicators that you are currently undergoing an infestation of adware.
- Have you noticed recently that your PC is running a lot more sluggishly than it was a couple of days ago? Perhaps programs are slow to open, web pages keep crashing, and when you do manage to spend any time online, your internet connection is frustratingly slow. This is a sure fire sign that you have something undesirable installed on your device. Adware can take its toll on your operating system thanks to its habit of installing a tracking component on your machine which monitors which websites you visit. Data about these visits is then recorded and sent back to the adware's programmer who will ensure that the adverts you see in the future are in alignment with your tastes and interests – thus increasing the chances of you clicking on them.
- Coupon Titan also negatively interact with any other programs you have installed on your computer, which not only makes them load slowly but can destabilize your system. And that can mean you are vulnerable to attack by something even more serious, as other types of malware will take advantage of the instability in your security.
- Suddenly found that you have a lot less space than you thought on your hard drive? That will be the adware. Thanks to its existence on your system, it can use up valuable memory space, leaving you struggling to find somewhere to store the documents, files, programs and photos that you DO want to keep.
Written by Michael Kaur, http://deletemalware.blogspot.com
"Ads by Coupon Titan" Removal Guide:
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove Coupon Titan related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
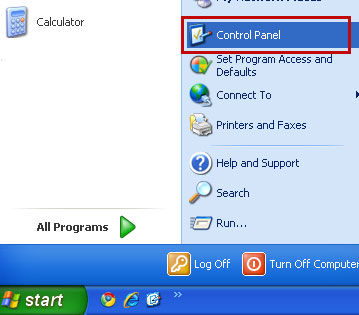
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
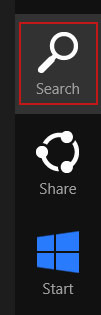
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
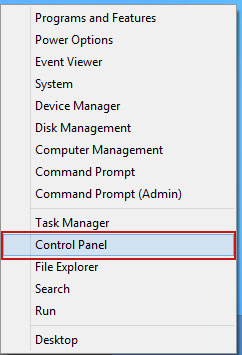
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
- Coupon Titan
- GoSave
- Active Discount
- AdCoupon
- and any other recently installed application
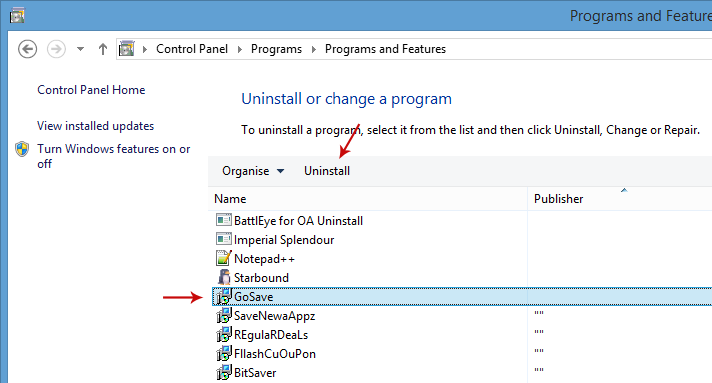
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove Coupon Titan related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
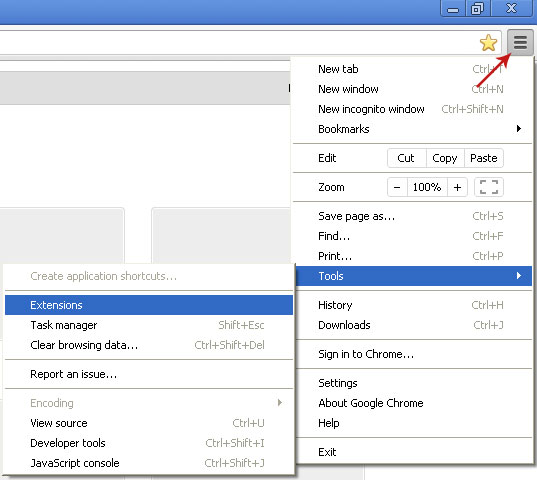
2. Click on the trashcan icon to remove Coupon Titan, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove Coupon Titan related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
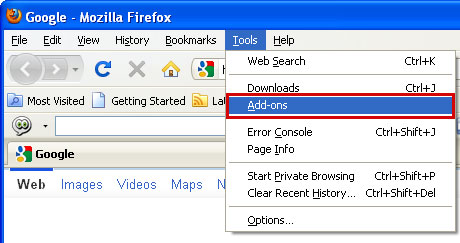
2. Select Extensions. Click Remove button to remove Coupon Titan, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove Coupon Titan related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
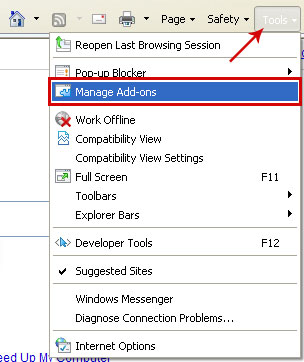
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
Remove URL:Mal svchost.exe Malware Infection (Uninstall Guide)
Posted on 11:27
by Malik korrich
You may well have wondered what URL:Mal malware in svchost.exe is, how it can infect your PC, what it does once it is present – and possibly you've also questioned how to get rid of it. Here we are going to take a closer look at the oddly monikered malicious software so that you can learn a little more about it and be better prepared when it comes to protecting yourself from an attack. Also, if you are reading this, chances are that your computer is already infected by URL:Mal svchost.exe. In such case, please follow the steps in the removal guide below.
You might take a certain pride in your ability to stay safe when you're online. You choose passwords that are hard to crack, you have a decent anti-virus program installed, you don't open email attachments from spammers and you don't tell the world on social media when you're going to be out of town and your home left empty. Well we are sorry to be the bearers of bad news because if you've been infected by the URL:Mal svchost.exe malware, your copy sheet will be well and truly blotted, as you will have been responsible for triggering the infestation.
And if you're wondering, that is precisely how this malware gets its name – from the Web Shield detection algorithms. A malicious website (or a file) is usually an object which is detected when a malicious file installed on your computer tries to connect to a remote web server controlled by cyber criminals. URL:Mal part simply means that the website is malicious and has to be blocked. Now, the svchost.exe is actually a genuine Windows file used by malware to avoid anti-virus detection. The malware uses dll injection to add its code to a genuine Windows file, in this case svchost.exe, and thus avoid Windows firewall and anti-virus detection. Obviously, you can't just delete svchost.exe from the system. You won't remove the malware and besides Windows probably won't let you to remove it anyway. You need tools for URL:Mal svchost.exe detection and cleaning. That's the only way to remove this malware without corrupting Windows system files.
Just like the giant horse that posed as something harmless, URL:Mal svchost.exe will similarly try and trick you into thinking that there is nothing wrong with it. Fall for its wiles and you'll accept it beyond your computer's defenses so that it can wreak havoc on your system from the inside.
So how do you know if you are in the presence of URL:Mal svchost.exe? The malware can manifest itself as a pop-up window that a former malware infection has left on your PC. You can also be at risk if you download a file, app or some software that has been compromised and packaged with a Trojan. Yet other Horses may be hidden in a link or an attachment sent via email or instant messenger.
Wondering what this malware infection can do? It can create instability in your operating system and problems on your hard drive, and it can also corrupt files, programs and data. You might suddenly find that you cannot access files, apps or software as well. In short, URL:Mal infections are a nightmare so if something is trying to tempt you into downloading it, stop and wonder why before you do some real damage.
To remove URL:Mal svchost.exe malware and other threats from the same malware family that may have been installed on your computer, please follow the removal guide below. If you have questions, leave a down comment below. I will be more than happy to help you. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download recommended anti-malware software and run a full system scan. It will detect and remove this infection from your computer.
NOTE: If you are using Internet Explorer and can't download anti-malware software because "Your current security settings do not allow this file to be downloaded" then please reset IE security settings and try again.
2. Download and run TDSSKiller. Press the button Start scan for the utility to start scanning.
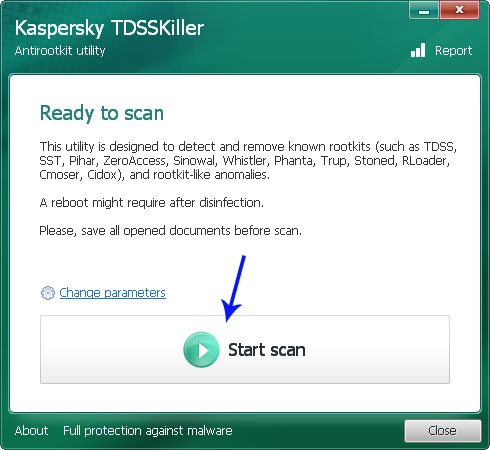
3. Wait for the scan and disinfection process to be over. Then click Continue. Please reboot your computer after the disinfection is over.
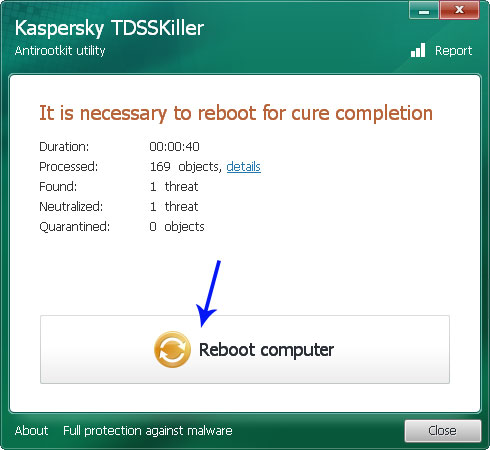
You might take a certain pride in your ability to stay safe when you're online. You choose passwords that are hard to crack, you have a decent anti-virus program installed, you don't open email attachments from spammers and you don't tell the world on social media when you're going to be out of town and your home left empty. Well we are sorry to be the bearers of bad news because if you've been infected by the URL:Mal svchost.exe malware, your copy sheet will be well and truly blotted, as you will have been responsible for triggering the infestation.
And if you're wondering, that is precisely how this malware gets its name – from the Web Shield detection algorithms. A malicious website (or a file) is usually an object which is detected when a malicious file installed on your computer tries to connect to a remote web server controlled by cyber criminals. URL:Mal part simply means that the website is malicious and has to be blocked. Now, the svchost.exe is actually a genuine Windows file used by malware to avoid anti-virus detection. The malware uses dll injection to add its code to a genuine Windows file, in this case svchost.exe, and thus avoid Windows firewall and anti-virus detection. Obviously, you can't just delete svchost.exe from the system. You won't remove the malware and besides Windows probably won't let you to remove it anyway. You need tools for URL:Mal svchost.exe detection and cleaning. That's the only way to remove this malware without corrupting Windows system files.
Just like the giant horse that posed as something harmless, URL:Mal svchost.exe will similarly try and trick you into thinking that there is nothing wrong with it. Fall for its wiles and you'll accept it beyond your computer's defenses so that it can wreak havoc on your system from the inside.
So how do you know if you are in the presence of URL:Mal svchost.exe? The malware can manifest itself as a pop-up window that a former malware infection has left on your PC. You can also be at risk if you download a file, app or some software that has been compromised and packaged with a Trojan. Yet other Horses may be hidden in a link or an attachment sent via email or instant messenger.
Wondering what this malware infection can do? It can create instability in your operating system and problems on your hard drive, and it can also corrupt files, programs and data. You might suddenly find that you cannot access files, apps or software as well. In short, URL:Mal infections are a nightmare so if something is trying to tempt you into downloading it, stop and wonder why before you do some real damage.
To remove URL:Mal svchost.exe malware and other threats from the same malware family that may have been installed on your computer, please follow the removal guide below. If you have questions, leave a down comment below. I will be more than happy to help you. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
URL:Mal svchost.exe Removal Guide:
1. First of all, download recommended anti-malware software and run a full system scan. It will detect and remove this infection from your computer.
NOTE: If you are using Internet Explorer and can't download anti-malware software because "Your current security settings do not allow this file to be downloaded" then please reset IE security settings and try again.
2. Download and run TDSSKiller. Press the button Start scan for the utility to start scanning.
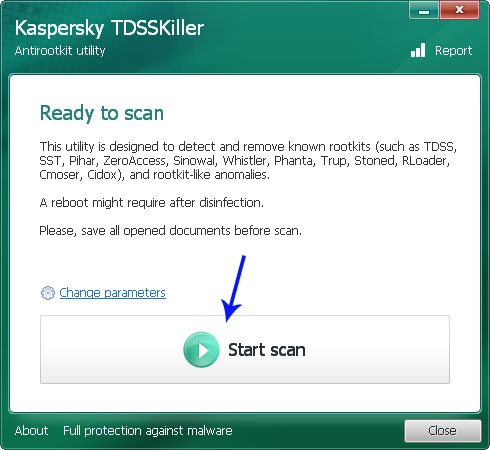
3. Wait for the scan and disinfection process to be over. Then click Continue. Please reboot your computer after the disinfection is over.
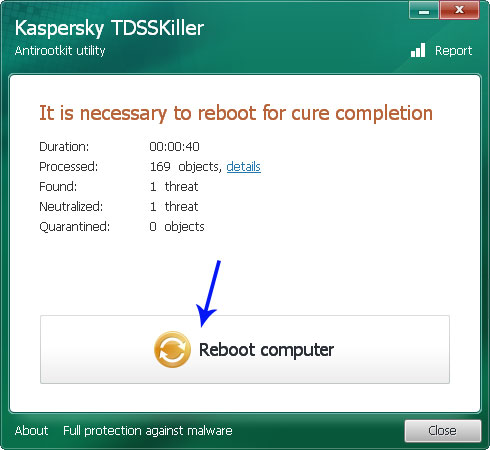
How to Check is a String is Palindrome in Java using Recursion
Posted on 08:58
by Malik korrich
In this tutorial, you will learn how to check if a string is a palindrome in Java using recursion. A String is nothing but a collection of characters e.g. "Java" and String literals are encoded in double quotes in Java. A String is said to be a palindrome if the reverse of String is equal to itself e.g. "aba" is a palindrome because the reverse of "aba" is also "aba", but "abc" is not a palindrome because the reverse of "abc" is "cba" which is not equal. Recursion means solving a problem by writing a function which calls itself. In order to check if String is a palindrome in Java, we need a function which can reverse the String. Once you have original and reversed String, all you need to do is check if they are equal to each other or not. If they are equal then String is palindrome or not. You can write this reverse() function by using either for loop or by using recursion.
Read more »How to create HTTP Server in Java - ServerSocket Example
Posted on 07:20
by Malik korrich
Java has a very good networking support, allows you to write client server application by using TCP Sockets. In this tutorial, we will learn how to create a simple HTTP Server in Java, which can listen HTTP request on a port let's say 80 and can send response to client. Being an HTTP Server, you can connect to it using your browser e.g. Chrome, Firefox or Internet Explorer. Though HTTP is ubiquitous and present everywhere, Java doesn't have a dedicated API to create and parse HTTP request, there is no in built HTTP client library in JDK. Though there is no short of good open source library e.g. you can use Jsoup to parse HTML and can use Apache HttpClient library for sending GET and POST request right from your Java program. By the way, for those who wants to master network programming in Java, I suggest to read Java Network Programming, 4th Addition by Harold, Elliotte Rusty, its very comprehensive and not only covers both TCP/IP and UDP protocols, which are backbone of internet but also dive deep into the HTTP protocol, including REST, HTTP headers, and cookies. Book is very focused on practical and you will find lot of interesting example related to common networking task e.g. writing multi-threaded servers, using non blocking IO and using low level socket classes.
Read more »lundi 22 juin 2015
Remove "Ads by couponing" Adware (Uninstall Guide)
Posted on 11:24
by Malik korrich
Couponing adware: just one thing in a long list of dangerous software that could end up causing very serious issues for you if you are unlucky enough to be infected by it. This adware can cause some very real issues, affecting not just your PC's operating speed but that of your internet connection too. It can also cause instability with the other programs or applications that you have running on your device – which can create security issues. This in turn has the knock on effect of weakening your defense and making it easier for even nastier forms of malware to attack you. And considering these programs may be designed to steal your data or identity and empty out your bank account, it makes sense to protect yourself from the so called 'nice guy' of the malicious software family.
What is couponing adware?
It is a type of software that is installed on your computer to show you adverts. Usually, these ads have labels "Ads by couponing", etc. In the majority of cases, couponing adverts will be for a product or a service that you have recently been looking at online. How does the adware know what you've been browsing? Well that's because it has been designed to also track your user habits: it will monitor which websites you are looking at and the content on the pages within.
Is couponing adware the same as spyware?
If you're thinking that this sounds a little like that other intrusive malware known as spyware you would not be far wrong. Spyware also tracks your computer use but for even scarier reasons. It can still see which websites you visit but it has additional 'features' and can also monitor which keys you type. It then logs this information and sends it back to the programmer who can use it at their will. And when you take into consideration that we all type personal data such as passwords and credit card numbers into our computers, often several times a day it gets even more worrying.
Here's an example of "Ads by couponing" adverts injected above Google search results.
Spyware is truly dangerous and because adware can weaken your security, as noted above, it means that you really should protect yourself from adware, even if you aren't worried about a third party tracking which websites you visit.
So how do you do that? Here are a few of the simple precautions you could, and should, take:
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove Couponing related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
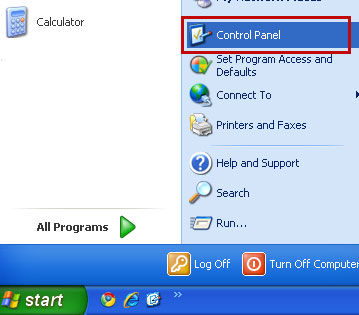
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
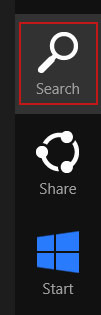
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
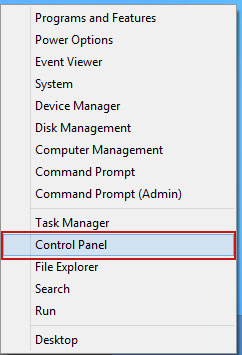
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
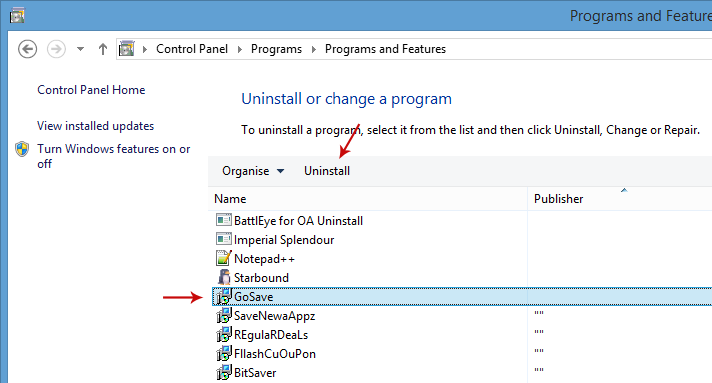
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove Couponing related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
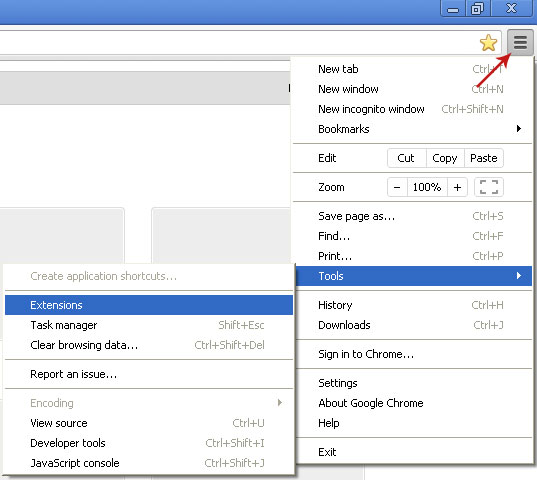
2. Click on the trashcan icon to remove couponing, Active Discount, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove Couponing related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
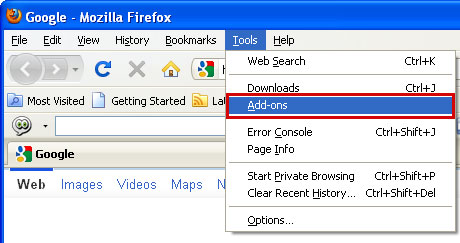
2. Select Extensions. Click Remove button to remove couponing, Active Discount, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove Couponing related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
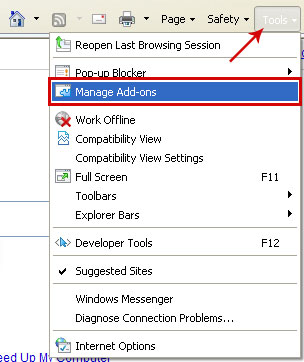
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
What is couponing adware?
It is a type of software that is installed on your computer to show you adverts. Usually, these ads have labels "Ads by couponing", etc. In the majority of cases, couponing adverts will be for a product or a service that you have recently been looking at online. How does the adware know what you've been browsing? Well that's because it has been designed to also track your user habits: it will monitor which websites you are looking at and the content on the pages within.
Is couponing adware the same as spyware?
If you're thinking that this sounds a little like that other intrusive malware known as spyware you would not be far wrong. Spyware also tracks your computer use but for even scarier reasons. It can still see which websites you visit but it has additional 'features' and can also monitor which keys you type. It then logs this information and sends it back to the programmer who can use it at their will. And when you take into consideration that we all type personal data such as passwords and credit card numbers into our computers, often several times a day it gets even more worrying.
Here's an example of "Ads by couponing" adverts injected above Google search results.
Spyware is truly dangerous and because adware can weaken your security, as noted above, it means that you really should protect yourself from adware, even if you aren't worried about a third party tracking which websites you visit.
So how do you do that? Here are a few of the simple precautions you could, and should, take:
- Downloading an app or program? Couponing adware is often bundled with free programs or peer to peer files. However it is normally mentioned in the small print. Therefore take the time to read this and make sure your configure boxes correctly as these may already be checked or unchecked so as to automatically install the adware.
- Never download files in emails from unknown senders.
- Install pop-up blockers and use a firewall.
- Close unknown pop-up windows by clicking the 'X' in the corner. Clicking 'Close' may trigger a further installation.
- Make sure you have a decent, up to date anti-malware program installed on your PC
Written by Michael Kaur, http://deletemalware.blogspot.com
"Ads by couponing" Removal Guide:
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove Couponing related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
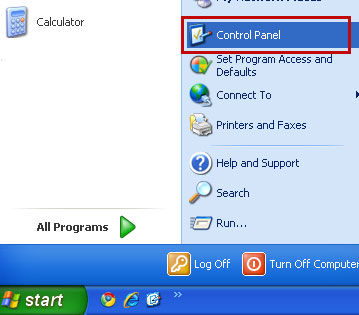
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
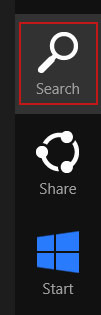
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
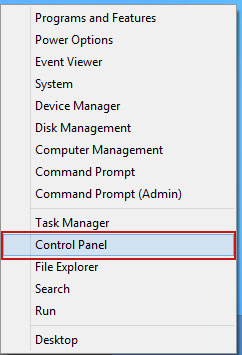
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
- Couponing
- GoSave
- Active Discount
- SaveNewaAppz
- and any other recently installed application
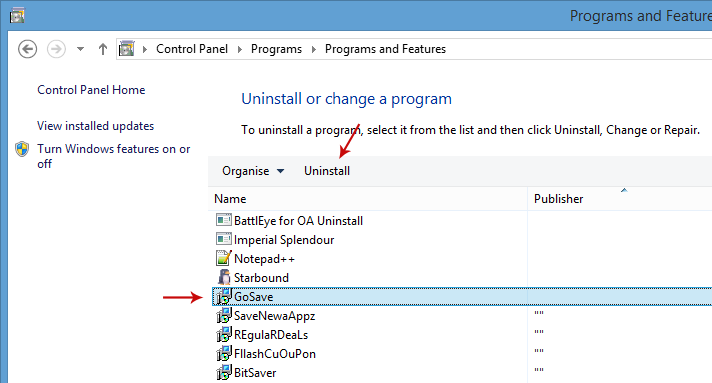
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove Couponing related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
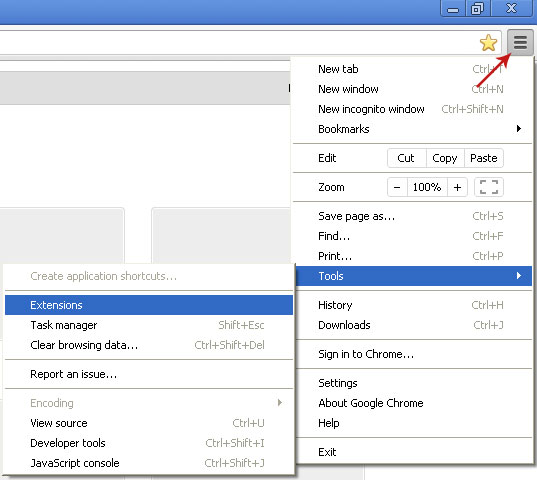
2. Click on the trashcan icon to remove couponing, Active Discount, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove Couponing related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
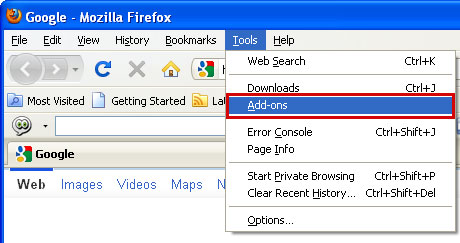
2. Select Extensions. Click Remove button to remove couponing, Active Discount, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove Couponing related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
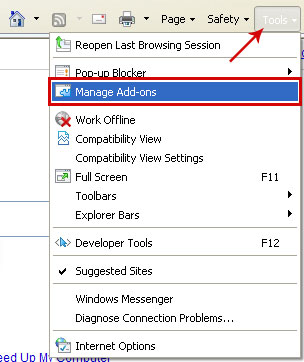
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
Remove "Ads by HugeApps" Adware (Uninstall Guide)
Posted on 10:30
by Malik korrich
If you are reading this, it is likely that you getting "Ads by HugeApps" advertisements on your web browser. Have you heard about HugeApps adware? Despite that, or even if you haven't heard of it, the chances are very high that once explained, you will realize that it is something that you see every day – at least when you are connected to the internet. And you may have even been infected by this adware, even if you didn't know about it.
In this article we are going to explore exactly what HugeApps adware is. Plus I'll tell you just how it infects your computer, as well as the steps you can take to ensure that you don't become a victim of this adware infection and its annoying "Ads by HugeApps" pop-ups the next time a programmer tries to install their product on your device.
How HugeApps adware gets on to your computer
It would be misleading of me to tell you that adware is something which sneaks up on you and then installs itself completely without your knowledge. And that is because, as with some other types of malware, YOU are actually responsible for the download and installation. Okay so it is fair to say that HugeApps adware does act in rather an underhand manner – or at least its developer does. And that;s because it normally comes bundled with another software program, app or file that you have opened or downloaded. In a far smaller percentage of cases it can also be stored in the cookies found on certain websites.
So what happens is that you are downloading or installing an upgrade, or a new program or app. If that file has adware packaged with it, if you don't read the License Agreement carefully and make sure you know exactly what you are installing, you will also end up installing the adware. So what happens if you discover a mention of an add-on program in the small print? You should either abort the download, or make sure you have the check boxes correctly configured so that the adware is not automatically installed.
Why do programmers use HugeApps adware?
Adware is, as the name suggests, advertising software and it has been created to generate an income for the company or brand who is marketing their product, and for the adware's programmer. And because having HugeApps adware installed on your computer is often very annoying, this is why the programmer goes to some length to hide it and hope that you download it unwittingly.
As well as being annoying, it can also...
Slow your computer's operating system and internet connection down thanks to a tracking component that it also installs on your PC. This monitors which websites you visit so that the programmer can customize which HugeApps adverts you see. And if this gross invasion of your privacy wasn't bad enough, adware can also adversely affect other programs that you have installed, causing instabilities with your functioning and therefore leaving your security solution unstable and open to breaches.
If it's already too late and your computer is infected with this adware and its relentless HugeApps advertisements, please follow the steps on the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove HugeApps related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
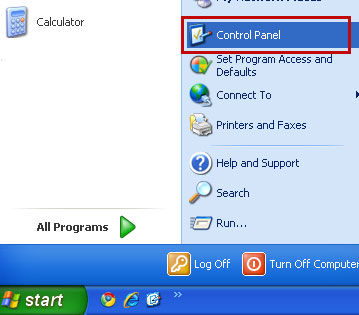
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
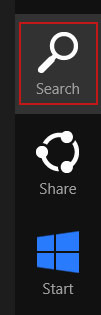
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
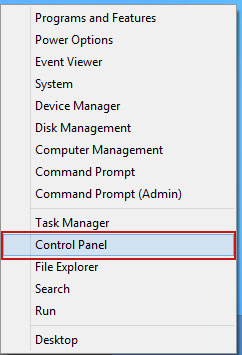
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
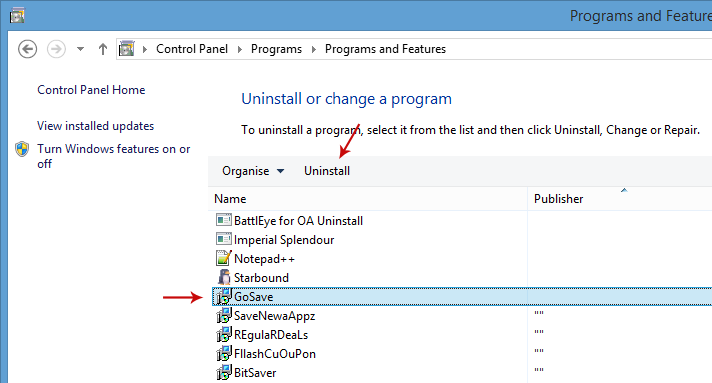
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove HugeApps related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
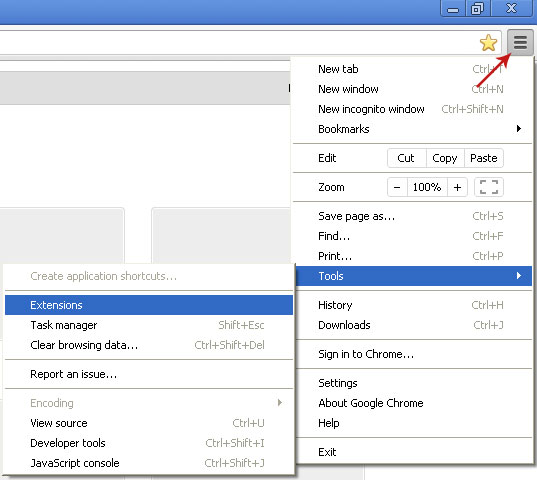
2. Click on the trashcan icon to remove HugeApps, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove HugeApps related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
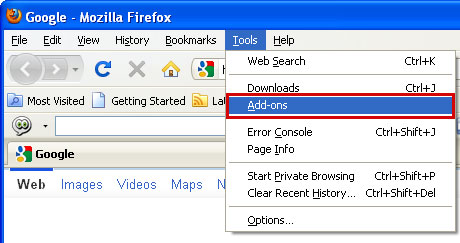
2. Select Extensions. Click Remove button to remove HugeApps, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove HugeApps related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
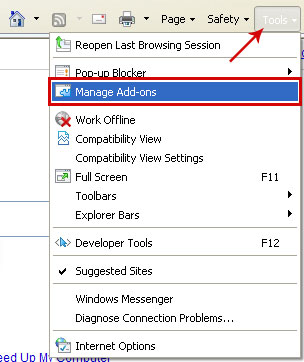
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
In this article we are going to explore exactly what HugeApps adware is. Plus I'll tell you just how it infects your computer, as well as the steps you can take to ensure that you don't become a victim of this adware infection and its annoying "Ads by HugeApps" pop-ups the next time a programmer tries to install their product on your device.
How HugeApps adware gets on to your computer
It would be misleading of me to tell you that adware is something which sneaks up on you and then installs itself completely without your knowledge. And that is because, as with some other types of malware, YOU are actually responsible for the download and installation. Okay so it is fair to say that HugeApps adware does act in rather an underhand manner – or at least its developer does. And that;s because it normally comes bundled with another software program, app or file that you have opened or downloaded. In a far smaller percentage of cases it can also be stored in the cookies found on certain websites.
So what happens is that you are downloading or installing an upgrade, or a new program or app. If that file has adware packaged with it, if you don't read the License Agreement carefully and make sure you know exactly what you are installing, you will also end up installing the adware. So what happens if you discover a mention of an add-on program in the small print? You should either abort the download, or make sure you have the check boxes correctly configured so that the adware is not automatically installed.
Why do programmers use HugeApps adware?
Adware is, as the name suggests, advertising software and it has been created to generate an income for the company or brand who is marketing their product, and for the adware's programmer. And because having HugeApps adware installed on your computer is often very annoying, this is why the programmer goes to some length to hide it and hope that you download it unwittingly.
As well as being annoying, it can also...
Slow your computer's operating system and internet connection down thanks to a tracking component that it also installs on your PC. This monitors which websites you visit so that the programmer can customize which HugeApps adverts you see. And if this gross invasion of your privacy wasn't bad enough, adware can also adversely affect other programs that you have installed, causing instabilities with your functioning and therefore leaving your security solution unstable and open to breaches.
If it's already too late and your computer is infected with this adware and its relentless HugeApps advertisements, please follow the steps on the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
"Ads by HugeApps" Removal Guide:
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this infection from your computer. You may then follow the manual removal instructions below to remove the leftover traces of this malware. Hopefully you won't have to do that.
2. Remove HugeApps related programs from your computer using the Add/Remove Programs control panel (Windows XP) or Uninstall a program control panel (Windows 7 and Windows 8).
Go to the Start Menu. Select Control Panel → Add/Remove Programs.
If you are using Windows Vista or Windows 7, select Control Panel → Uninstall a Program.
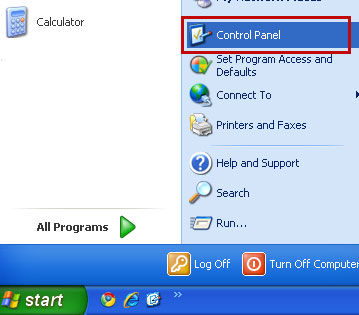
If you are using Windows 8, simply drag your mouse pointer to the right edge of the screen, select Search from the list and search for "control panel".
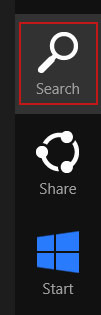
Or you can right-click on a bottom left hot corner (formerly known as the Start button) and select Control panel from there.
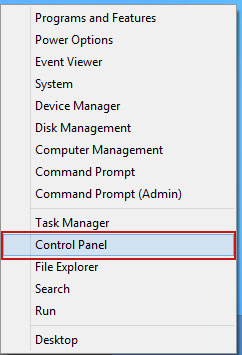
3. When the Add/Remove Programs or the Uninstall a Program screen is displayed, scroll through the list of currently installed programs and remove the following:
- HugeApps
- GoSave
- Active Discount
- AdCoupon
- and any other recently installed application
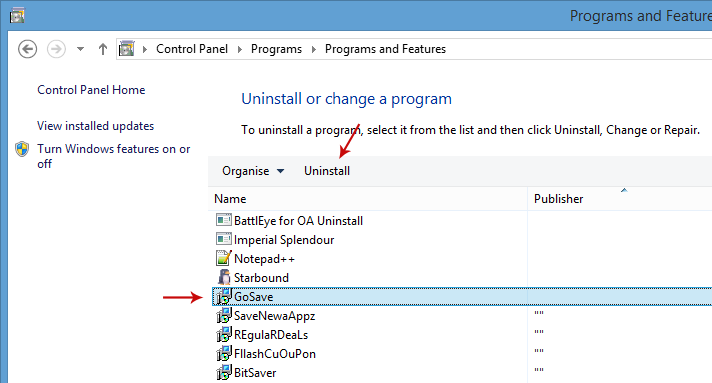
Simply select each application and click Remove. If you are using Windows Vista, Windows 7 or Windows 8, click Uninstall up near the top of that window. When you're done, please close the Control Panel screen.
Remove HugeApps related extensions from Google Chrome:
1. Click on Chrome menu button. Go to Tools → Extensions.
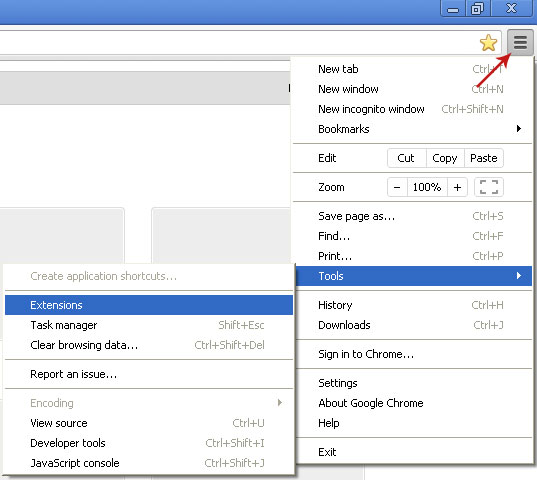
2. Click on the trashcan icon to remove HugeApps, AdCoupon, MediaPlayerV1, Gosave, HD-Plus 3.5 and other extensions that you do not recognize.
If the removal option is grayed out then read how to remove extensions installed by enterprise policy.

Remove HugeApps related extensions from Mozilla Firefox:
1. Open Mozilla Firefox. Go to Tools → Add-ons.
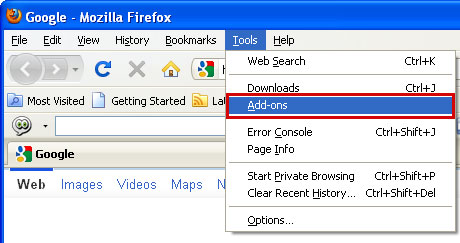
2. Select Extensions. Click Remove button to remove HugeApps, AdCoupon, Gosave, MediaPlayerV1, HD-Plus 3.5 and other extensions that you do not recognize.
Remove HugeApps related add-ons from Internet Explorer:
1. Open Internet Explorer. Go to Tools → Manage Add-ons. If you have the latest version, simply click on the Settings button.
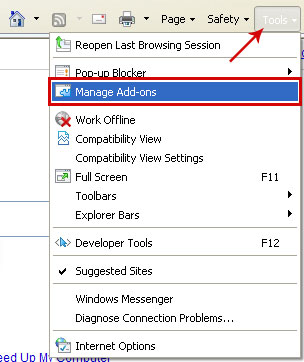
2. Select Toolbars and Extensions. Click Remove/Disable button to remove the browser add-ons listed above.
Remove MSIL/Injector.YT Trojan (Uninstall Guide)
Posted on 09:22
by Malik korrich
MSIL/Injector.YT - another Trojan where the clue is in the name. Expect an influx of malware, ransomware, and spyware to be installed on your PC if you've been hit by one of these. It uses DLL injection to run malicious code within the address space of another process. This technique is very common with modern malware, especially Trojan horses and spyware. Even if you only know one thing about malicious software, the chances are that you know that generally speaking it infects your computer by hiding itself in an attachment in a spam email, or with another program or file download. Spam emails and their attachments are something that we all know we need to be extremely wary of and avoid opening at all costs, but what about downloads? Here, unfortunately, it is not quite so crystal clear. After all, spam is spam, but downloaded programs, files and torrents are another thing altogether and such a broad category that it is impossible to say what you should and shouldn't download.
And that's because malware and other internet pests, such as MSIL/Injector.YT can come packaged with (as you would assume) X rated adult content just as easily as they can with an innocent instant messenger app, or even something known and trusted such as an Adobe product or internet phone solution such as Skype. Hell, even that upgrade to your trusted anti-virus program can be at risk. Whether you are installing, downloading or upgrading, variants of MSIL/Injector.YT trojan are not fussy and will use any means to install themselves on your computer.
But I can't live without my software tools
Whoa there – don't panic as no one is suggesting that we give up downloading software and apps for good. Modern life is too far gone for a step in that direction! But there are things that you now need to take into consideration to avoid the dangers and nuisance caused by MSIL/Injector.YT trojan. And for the most part that means staying one step ahead of the latest threat doing the rounds, educating yourself, knowing when your computer is running differently to before, and being careful when you download or upgrade anything.
If all that sounds a little daunting, don't worry, as there are some simple (and free!) ways that you can avoid, at least to the best of your abilities, downloading a variant of MSIL/Injector.YT trojan alongside the program, file or app that you do want.
Easy habits that you should adopt now if you want to avoid being infected by this Trojan horse
When you download a file or program, make sure you read the software license agreement properly.
Make sure your PC is running on the latest operating system by installing the newest patches as released by Microsoft and that all the programs (including of course your security software) are on the current version.
Make sure your anti-virus is up to date
Don't open email attachments from unknown senders and even if you do know them still scan files before downloading them
How do I remove MSIL/Injector.YT Trojan?
It's one of many deeply embedded malware which can be difficult to remove. I definitely do not recommend manual removal. You probably won't find it and even if you would remember that it injects its code into genuine Windows files. Removing any of these can make your system unstable and cause crashes. The best thing you can do is use the removal tools give below. The more the better because it's a rather sophisticated malware and not all tools are able to detect and permanently remove it from the system. To remove this Trojan horse from your computer, please follow the steps in the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this Trojan from your computer. You may then run the second removal tool given below to remove the leftover traces of this malware. Hopefully you won't have to do that.
NOTE: If you are using Internet Explorer and can't download anti-malware software because "Your current security settings do not allow this file to be downloaded" then please reset IE security settings and try again.
2. Download and run TDSSKiller. Press the button Start scan for the utility to start scanning.
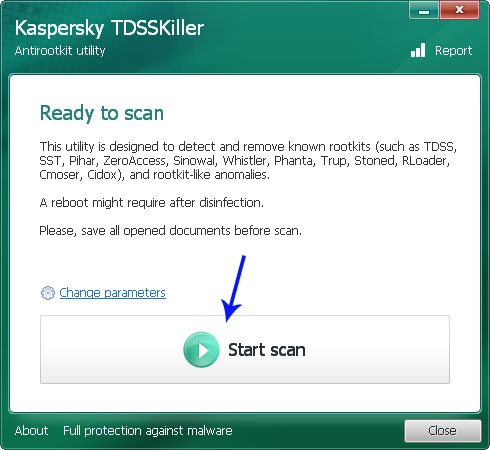
3. Wait for the scan and disinfection process to be over. Then click Continue. Please reboot your computer after the disinfection is over.
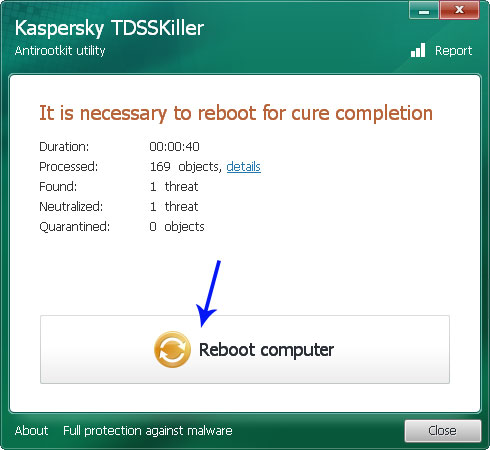
And that's because malware and other internet pests, such as MSIL/Injector.YT can come packaged with (as you would assume) X rated adult content just as easily as they can with an innocent instant messenger app, or even something known and trusted such as an Adobe product or internet phone solution such as Skype. Hell, even that upgrade to your trusted anti-virus program can be at risk. Whether you are installing, downloading or upgrading, variants of MSIL/Injector.YT trojan are not fussy and will use any means to install themselves on your computer.
But I can't live without my software tools
Whoa there – don't panic as no one is suggesting that we give up downloading software and apps for good. Modern life is too far gone for a step in that direction! But there are things that you now need to take into consideration to avoid the dangers and nuisance caused by MSIL/Injector.YT trojan. And for the most part that means staying one step ahead of the latest threat doing the rounds, educating yourself, knowing when your computer is running differently to before, and being careful when you download or upgrade anything.
If all that sounds a little daunting, don't worry, as there are some simple (and free!) ways that you can avoid, at least to the best of your abilities, downloading a variant of MSIL/Injector.YT trojan alongside the program, file or app that you do want.
Easy habits that you should adopt now if you want to avoid being infected by this Trojan horse
When you download a file or program, make sure you read the software license agreement properly.
Make sure your PC is running on the latest operating system by installing the newest patches as released by Microsoft and that all the programs (including of course your security software) are on the current version.
Make sure your anti-virus is up to date
Don't open email attachments from unknown senders and even if you do know them still scan files before downloading them
How do I remove MSIL/Injector.YT Trojan?
It's one of many deeply embedded malware which can be difficult to remove. I definitely do not recommend manual removal. You probably won't find it and even if you would remember that it injects its code into genuine Windows files. Removing any of these can make your system unstable and cause crashes. The best thing you can do is use the removal tools give below. The more the better because it's a rather sophisticated malware and not all tools are able to detect and permanently remove it from the system. To remove this Trojan horse from your computer, please follow the steps in the removal guide below. If you have any questions, please leave a comment down below. Good luck and be safe online!
Written by Michael Kaur, http://deletemalware.blogspot.com
MSIL/Injector.YT Trojan Removal Guide:
1. First of all, download anti-malware software and run a full system scan. It will detect and remove this Trojan from your computer. You may then run the second removal tool given below to remove the leftover traces of this malware. Hopefully you won't have to do that.
NOTE: If you are using Internet Explorer and can't download anti-malware software because "Your current security settings do not allow this file to be downloaded" then please reset IE security settings and try again.
2. Download and run TDSSKiller. Press the button Start scan for the utility to start scanning.
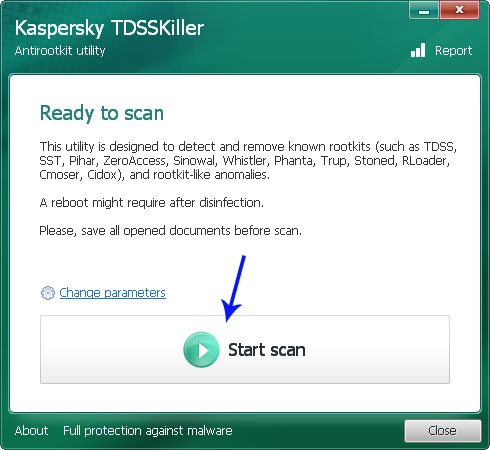
3. Wait for the scan and disinfection process to be over. Then click Continue. Please reboot your computer after the disinfection is over.
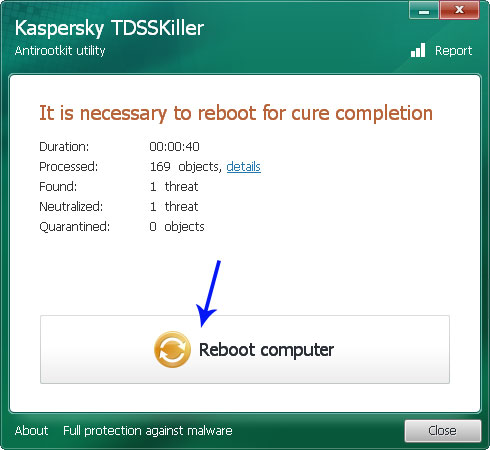
How to pause Thread in Java using Sleep() and TimeUnit Example
Posted on 08:42
by Malik korrich
There are multiple ways to pause or stop the execution of currently running thread, but putting the thread into sleep state using Thread.sleep() method is the right way to introduce pause. Some people would say, why not use wait and notify?. Using those methods just for pausing thread is not good. Those are the tools for a conditional wait and they don't depend on upon time. A thread blocked using wait() will remain to wait until the condition on which it is waiting is changed. Yes, you can put timeout there but the purpose of wait() method is different, they are designed for inter-thread communication in Java. By using sleep() method, you pause the current for some given time. You should never use sleep() in place of wait() and notify() and vice-versa. There is another reason why to wait and notify should not be used to pause the thread, a they need lock. You can only call them from a synchronized method or block and acquire and release a lock is not cheap.
Read more »2 Ways to Parse CSV Files in Java - BufferedReader vs Apache
Posted on 07:00
by Malik korrich
In last tutorial, you have learned how to parse Excel file in Java and in this Java tutorial, you will learn how to parse CSV file in Java. You can directly parse CSV file in Java without using any third party library, because ultimately its a text file and you can use BufferedReader to read it, but you can also take advantage of good open source library like Apache commons CSV to parse comma separated values. These library makes developer's life easy and provides rich functionality to parse various CSV formats. In real programming world, CSV or comma separated files are used for variety of purpose, including for transporting data from one system to another e.g. FX rates, importing and exporting records from database etc. In CSV files entries are separated by comma, and it may or may not contain header. There are many ways to parse or read CSV files in Java, and if you need to do it on your project, its better not to reinvent the wheel and choose commons csv, but for learning purpose, it's good to know how to do it without using third party library.
Read more »dimanche 21 juin 2015
How Programmers Life Can Bring a Smile to Your Face
Posted on 14:32
by Malik korrich
I stumbled upon the site thecodinglove.com and just loved it . I want to use the images and quotes to share their awesome work with you guys . Guess what! they have granted the permission . Although there are thousand of images to choose from the website . I am sharing which relates the most of programmer's lives.This is hilarious, so, pause your day and bring a smile to your face.
















When a newbie suggests to add a new feature to project
Fixing minor bugs
When I'm told that the module on which I have worked all the week will never be used
When I try to fix a bug at 3 in the morning
When I have no choice but to work directly on the Production server
When people tell me PHP is not a real programming language
Backend developer working on the user interface
"Just deploy it, it’s friday!"
When I'm told that my code is broken in production
When I show the boss that I have finally fixed this bug
When the project manager enters the office
When I apply a new CSS for the first time
When that nasty bug comes back again
When sysadmin finally gives us the root access
When the app goes into beta and the first bug reports arrive
Classic development cycle.
When the boss is looking for someone to urgently fix a difficult bug
Want to have more fun
Click here for part 2
Share this post and give a SMILE :) to someone else :D